Using Tailwind CSS with React and CSS-in-JS
If you've looked into adding Tailwind CSS to your React project, chances are you've seen the official installation guides, but what if you're already using Emotion, or styled-components?
Sure, you could probably try some funky combination of className and styled components such as:
const MyStyledDiv = styled.div` color: red;`;
const MyComponent = () => <MyStyledDiv className="mt-4 flex" />;
Assuming that would even work...
But what if you could use Tailwind classnames with your current CSS-in-JS configuration?
Introducing twin.macro
twin.macro by Ben Rogerson lets you write Tailwind CSS inside your CSS-in-JS, lints your Tailwind classnames, and doesn't make your bundle bigger.
twin.macro gives you access to a few things:
a
tw
prop on JSX elements:import 'twin.macro';const MyDiv = () => <div tw="border hover:border-black" />;Tailwind class linting (this is the main reason I use this library):
✕ ml-7 was not foundTry one of these classes:ml-0 [0] / ml-1 [0.25rem] / ml-2 [0.5rem] / ml-3 [0.75rem] / ml-4 [1rem] / ml-5 [1.25rem] / ml-6 [1.5rem]ml-8 [2rem] / ml-10 [2.5rem] / ml-12 [3rem] / ml-16 [4rem] / ml-20 [5rem] / ml-24 [6rem] / ml-32 [8rem]ml-40 [10rem] / ml-48 [12rem] / ml-56 [14rem] / ml-64 [16rem] / ml-auto [auto] / ml-px [1px]The standard Styled Component API:
import tw from 'twin.macro';const MyDiv = tw.div`border hover:border-black`;// and cloning/styling other componentsconst PurpleDiv = tw(MyDiv)`border-purple-500`;The
css
prop:import tw from 'twin.macro';const Input = ({ hasHover }) => (<input css={[tw`border`, hasHover && tw`hover:border-black`]} />);
The library currently supports both Emotion and styled-components when using React, Preact, Create React App, Gatsby, Next.js, Snowpack, and more.
You'll want to see the Get started section of the README for instructions on how to install it for your particular combination of CSS-in-JS library and React framework.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
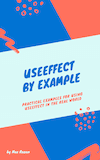