How to style react-select with styled-components or emotion
For customisable dropdowns, react-select is one of the most popular select dropdown pickers in Github.
In terms of CSS-in-JS solutions, styled-components and emotion are often the first options developers consider. They use the same API, which makes it a breeze to swap between the two.
How to use react-select and styled-components together:
import React from "react";import ReactSelect from "react-select";import styled from 'styled-components';
const MultiSelect = styled(ReactSelect)` &.Select--multi {
.Select-value { display: inline-flex; align-items: center; } }
& .Select-placeholder { font-size: smaller; }`
export (props) => <MultiSelect multi {...props} />
How to use react-select and emotion together:
import React from "react";import ReactSelect from "react-select";import styled from 'emotion';
const MultiSelect = styled(ReactSelect)` &.Select--multi {
.Select-value { display: inline-flex; align-items: center; } }
& .Select-placeholder { font-size: smaller; }`
export (props) => <MultiSelect multi {...props} />
It's really that easy to go between the two libraries.
You can also do the exact same thing with react-dates saving you from having those pesky global.css files several thousand lines long.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
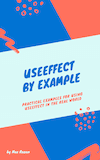