Keeping up with React Libraries
It's no secret React has a library discoverability problem.
While the number of stars in GitHub and weekly downloads in npm might be a good starting point for finding quality libraries, normally you have to wade through a lot of reddit, hacker news, dev.to and individual blog posts to find the best ones.
In this (continually updated) article, I'll be adding libraries (excluding component libraries, I track those here) worth talking about on a single page.
Table of Contents
3D
react-three-fiber is a React renderer for threejs on the web and react-native.
Chances are, if you've seen really cool 3D animations on a website, it was probably built in three.js - react-three-fiber gives you a way to tap into React while building your 3d scenes. There's also a Next.js + Tailwind starter worth looking into.
Accessibility
React Aria provides you with Hooks that provide accessibility for your components, so all you need to worry about is design and styling. Particularly useful for those building design systems.
Example usage - useButton:
import { useButton } from '@react-aria/button';
function Button(props) { let ref = React.useRef(); let { buttonProps } = useButton(props, ref);
return ( <button {...buttonProps} ref={ref}> {props.children} </button> );}
<Button onPress={() => alert('Button pressed!')}>Press me</Button>;
Animation
Animation adds soul to otherwise boring things. These libraries let you build the web app equivalent of Pixar's Intro Animation, but in React.
Both libraries have similar APIs and support spring physics over time-based animation, though Framer Motion seems to be used more often on GitHub.
Framer Motion is an animation and gesture library built by Framer. The added benefit of Framer Motion is that your designers can build animations in Framer, then hand-off designs to be accurately implemented using Framer's own library.
React Spring uses spring physics rather than time-based animation to animate your components. Relative to Framer Motion, React Spring has been under development for longer, with a greater number of open-source contributors.
Browser Features
Ever been asked to implement random features that someone on the product team saw on another website and thought was cool? These libraries save you time on building those features.
useClippy is a React hook that lets you read and write to your user's clipboard. Particularly useful for improving UX, letting you save your users from double clicking on your data fields, by providing them a button to copy values.
ReactPlayer is an awesome library that lets you embed video from major sources (YouTube, Facebook, Twitch, SoundCloud, and more), and define your own controls to the video.
React Toastify allows you to add fancy in-app notifications (like the "Message Sent" notification in Gmail) to your React app with only four additional lines of code.
Data Fetching Libraries
You might be wondering why you'd even need a data fetching library, when you could use useEffect
and fetch()
. The short answer is that these libraries also handle caching, loading and error states, avoiding redundant network requests, and much more.
You could spend hundreds of hours implementing these features in a Redux-like state manager, or just install one of these libraries.
React Query lets you request data from the same API endpoint (for example api/users/1
) across multiple components, without resulting in multiple network requests.
Similar to React Query (in fact, based on the same premise, see this issue for more info), SWR is another data fetching library worth checking out. SWR has the added security of being used by Vercel in production as part of their platform.
Data Visualisation
Everyone wants to have beautiful charts, but nobody wants to learn no complicated-ass D3
- Ronnie Coleman, probably
If you've ever used a popular charting library such as Recharts or Charts.js, you'll know it's surprisingly easy to reach the limits of what a charting library can do for you.
visx is different, in that it provides you with lower-level React components that are much closer to D3 than other charting libraries. This makes it easier to build your own re-usable charting library, or hyper-customised charts.
Forms
Forms suck. Take it from someone who once had to build a "smart" form with 26 possible fields to fill out - you want to pass off as much as possible to your form library, leaving you with only quick field names to enter.
React Hook Form is different to other form libraries, in that by default, you're not building controlled components and watching their state. This means your app's performance won't get slower as you add more fields to your form.
On top of that, it's probably one of the best documented libraries out there - every example has a CodeSandbox, making it easy to fork and try out your particular use case.
Mocking APIs
You could probably argue mocking libraries belong more under testing utilities, but they have added benefits during development too. While not specifically tied to React, they can be extremely useful when you want dynamic responses from your mocked endpoints.
MSW is a library that lets you mock both REST and GraphQL APIs, that uses service workers to generate actual network requests.
Why is that cool?
You can actually use MSW as part of your development workflow regardless of technology choice, not only while testing! It's so cool that Kent C. Dodds wrote a whole article about it.
In short, you can use MSW to experiment with building frontend features before the backend is ready AND mock your backend for tests.
State Management
There's been a fair bit of innovation in state management since the early days of Redux, it's worth taking a look again if you're interested in using global state.
Jotai describes itself as a primitive state management solution for React, and a competitor to Recoil. It's quite minimalist, meaning less API to learn, and if you understand React's useState
hook, you'll probably understand Jotai's useAtom
hook.
Mobx is a global state management library that requires a bit of a mental model shift to get used to. Once you get it though, Mobx provides a solution to state management and change propagation without the boilerplate.
Recoil is a state management library - think Redux meets React Hooks, minus the boilerplate.
Redux Toolkit (or RTK), is the official, opinionated way to manage your state using Redux.
RTK greatly reduces the amount of boilerplate necessary for using Redux, provides sensible defaults, and keeps the same immutable update logic that we know and love.
XState is a library that lets you formalise your React app as a finite state machine.
State machines aren't a particularly new concept, but developers have only recently started to realise that maybe our apps could be less buggy if we explicitly define the states they can be in, and the inputs required to transition between states.
XState also generates charts for you based on your app's xstate configuration, meaning your documentation will stay up to date as you code.
Zustand is another state management library, that aims to be simple and un-opinionated. The main difference is that you use hooks as the main way to fetch state, and doesn't require peppering your app with context providers.
Testing
React Testing Library (RTL) has become kind of a big deal for testing in React (and other libraries/frameworks). It's now the default testing library that comes with create-react-app.
RTL replaces Enzyme in your testing stack. While both libraries provide methods for rendering React components in tests, RTL exposes functions that nudge developers away from testing implementation details, and towards testing functionality.
Videos
Videos?! You ask? Why yes, dear reader. There are now libraries for making videos in React.
Remotion lets you make videos of your React components rendering - whether that involves fetching data from an API and displaying it, or showing cool animations.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
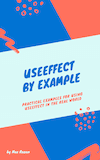