How to use SVGs in your React App
Trying to render an SVG in your React app, and getting errors? You're not alone - it's a relatively common issue.
There are two ways to do it, and both have tradeoffs.
Using <img>
tags, and passing your SVG's URL
Here's a basic example:
import React from 'react';import logoSrc from './logo.svg';
const MyLogo = () => { return <img src={logoSrc} />;};
The benefit of this approach is that your logo will not end up in your bundle, but rather exported as a static file when you run yarn build
(assuming you're using a standard webpack config, such as the one found in create-react-app).
This then gives you the option of aggressively caching icons that you know won't change.
You would typically use this approach for larger company logos on your marketing site, or for illustrations in your app.
Creating a React component, and passing props
The other option is to create a React component containing your SVG. Also known as "inlining" your SVG.
This done by pasting your raw svg
markup into a new React component.
There are a few ways to achieve this:
- Manually, byremoving/replacing all HTML props with the React equivalent, and adding
{...props}
to the mainsvg
element), - CLI via SVGR - a utility to automate this process
- Webpack config via SVGR
If you're using create-react-app, it already has SVGR's webpack config built-in, so you can already use your SVGs like React components:
import Star from './star.svg';const App = () => ( <div> <Star /> </div>);
Here's what a manually created SVG-based React component looks like:
import React from 'react';
export const DeleteIcon = (props) => ( <svg xmlns="http://www.w3.org/2000/svg" height="24px" viewBox="0 0 24 24" {...props} > <path d="M6 19c0 1.1.9 2 2 2h8c1.1 0 2-.9 2-2V7H6v12zM19 4h-3.5l-1-1h-5l-1 1H5v2h14V4z" /> <path d="M0 0h24v24H0z" fill="none" /> </svg>);
This approach lets you easily access props on your SVG icon. For example, changing the fill color:
<DeleteIcon fill="#fff" />
The downside being that your icons won't be as easily cached, so I would use this approach for smaller icons, such as the Material Design Icons.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
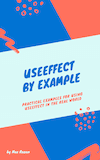