Guidelines for Deploying React
Figuring out how and where to deploy React can be pretty confusing. A common question for newcomers from other frameworks is "where does React run?", while others wonder what the best practices for deploying React to production are.
Let's clear up some of that confusion.
Table of ContentsOn static files
When using create-react-app, you trigger a React build by running npm run build
or yarn build
. The output you'd see looks like this:
We call these static files. When building client-side rendered apps (such as when using create-react-app), we only need to worry about where to host these files. Server-side rendering or SSR (running a server that generates the HTML the users see as they request it) also uses these files, but that's for a future article to cover.
Static files are awesome because you technically only need a file host to upload them to, in order to deploy your app. I say technically, because when we deploy to production, we typically also want a CDN and HTTPS to make our app fast and secure around the world.
So where does React "run"?
In short, if you're using create-react-app, React runs in your user's browser. Your static files get sent to them when they visit your app's URL.
Deploying React: DIY vs Platforms
There are two routes to deploying a React app on the internet:
DIY
Having worked for some old-school engineers who wanted to be able to see all of the magic before they trusted it, I basically learned how to build React apps while DIYing all of the infrastructure, and CI/CD.
Scaling the client-side part of React isn't particularly challenging: the key to not going offline during high load is a decent CDN, and good caching settings.
To DIY your React production setup, you'll want to:
- Choose a web host
- Choose a CDN provider (particularly one that provides free HTTPS)
- Choose a DNS provider
- Set up CI/CD
- (not mandatory, I've worked with very small teams that would just use package.json scripts to test and deploy their apps)
These days, I wouldn't particularly recommend small teams use the DIY approach. Your engineers' time is better spent building features or writing tests. God help you if you decide to deploy to Kubernetes before achieving product-market fit.
In my case with the old-school engineers, we had to support government clients, so we wanted to configure every part of the system by hand (via terraform), and to know exactly where our code was being deployed to. We ended up going with AWS to handle hosting (S3), CDN (CloudFront), DNS (Route 53), and CI/CD (Self-hosted Jenkins on AWS).
Years later, I would still pick AWS when going down the DIY route, the thing I'd change is opting for GitHub Actions or CircleCI for CI/CD.
Platforms
Platforms such as Netlify and Vercel are absolute magic compared to the DIY route.
Setting up a project with them is as simple as logging into the platform, authenticating to GitHub, picking a repo, double checking the default settings are correct, and hitting "Done".
For that, you automatically get:
- Hosting
- CDN with free HTTPS
- CI/CD
All that's left to do is point your DNS records to the platform.
You also get access to serverless functions, though limited compared to running directly on AWS.
The other day I setup useEffectByExample.com on Vercel. It took me about two hours total from completely new Next.js app to deployed production-grade React app. Roughly two minutes of which were spent setting up Vercel.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
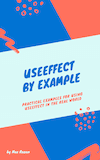