What is the difference between style-loader and mini-css-extract-plugin?
style-loader
style-loader
takes CSS you've imported in your JavaScript files, and injects them as <style></style>
tags into the DOM. It's particularly useful for inlining Critical CSS into the <head>
of your page.
If you decide to self-host your fonts instead of using Google Fonts, style-loader
can also help you inline your font declarations so the browser knows immediately what to do with the font files it downloads.
How do you use style-loader?
/* style.css */body { background: green;}
/* component.js */import './style.css';
/* webpack.config.js */module.exports = { module: { rules: [ { test: /\.css$/i, use: ['style-loader', 'css-loader'], }, ], },};
mini-css-extract-plugin
mini-css-extract-plugin
on the other hand, extracts your CSS into separate files.
It generates a CSS file for each JS file that imports CSS. It's more useful for CSS that you want to load asynchronously.
How do you use mini-css-extract-plugin?
/* webpack.config.js */const MiniCssExtractPlugin = require('mini-css-extract-plugin');module.exports = { plugins: [ new MiniCssExtractPlugin({ // Options similar to the same options in webpackOptions.output // all options are optional filename: '[name].css', chunkFilename: '[id].css', ignoreOrder: false, // Enable to remove warnings about conflicting order }), ], module: { rules: [ { test: /\.css$/, use: [ { loader: MiniCssExtractPlugin.loader, options: { // you can specify a publicPath here // by default it uses publicPath in webpackOptions.output publicPath: '../', hmr: process.env.NODE_ENV === 'development', }, }, 'css-loader', ], }, ], },};
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
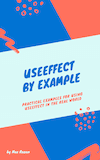