The Definitive Guide to Commonly Used Words in React
React has a lot of jargon and three-letter acronyms. Even as an intermediate React developer you might find yourself forgetting what certain things mean.
Got any React terms or expressions you'd like defined here? Feel free to message me via Email or BlueSky.
Table of Contents
Class Component
At its simplest, a Class Component is a component written using a JavaScript ES6 Class.
class Welcome extends React.Component { render() { return <h1>Hello, {this.props.name}</h1>; }}
Component
A Component is (most-commonly) a function that accepts props, and returns things to display in the browser.
See also: Class Component, Functional Component
CRA
CRA, or create-react-app, refers to a set of scripts open sourced by Facebook to make it easier to get started with your React app. It comes with its own opinions on webpack, testing, and linting.
CRA has become more popular recently as developers begin to realise maintaining their own webpack config and keeping related dependencies up to date isn't worth the time or energy.
Enzyme
Enzyme is a utility that renders React components in tests, and provides methods for querying their state and props.
Used in conjunction with Jest.
ESLint
ESLint is a program that is run to analyse your codebase, typically looking for ways to improve your code.
These "ways to improve your code" are typically distributed as a set of rules, such as eslint-config-react-app.
Some sets of rules are considered highly opinionated (such as eslint-config-airbnb), and aren't recommended for use as often.
Functional Component
A component written using a JavaScript function.
Thanks to the advent of Hooks, Functional components are considered "the way" components are written now.
Functional components can be written either by using an arrow function, or using the function
keyword.
Using an arrow function:
const Welcome = (props) => { return <h1>Hello, {props.name}</h1>;};
Using the function
keyword:
function Welcome(props) { return <h1>Hello, {props.name}</h1>;}
Flow
A type checker that can analyse types on any function, not just React components. It also checks your code at compile-time, rather than run-time.
Has fallen by the wayside due to TypeScript's meteoric rise to fame, thousands of unresolved GitHub issues, and sluggish performance.
Higher-order component
A fancy way of saying a component whose sole purpose is to wrap other components and pass props.
Links: docs
HOC
Hooks
Hooks let developers use state and other React features previously only available in class components, in functional components.
Links: docs | hooks reference | rules of hooks
Jest
Jest is a testing framework responsible for running your tests. Supports both Node and React (and other JS frameworks/languages).
Linting
Linting means to run a program that analyses your code for issues. Issues could mean syntax or style issues, as well as potential bugs.
The most commonly used linter in React is ESLint.
Props
Props are arguments you pass as input to a component in React.
PropTypes
PropTypes is a package (once part of React) that provides runtime type checking for your React components' props. It was one of the first ways to type-check React code.
Effectively superseded by Flow and TypeScript.
Usage (from the docs):
import React from 'react';import PropTypes from 'prop-types';
class MyComponent extends React.Component { render() { // ... do things with the props }}
MyComponent.propTypes = { // These are optional by default optionalArray: PropTypes.array, optionalFunc: PropTypes.func, optionalNumber: PropTypes.number, optionalString: PropTypes.string, requiredFunc: PropTypes.func.isRequired,
// A value of any data type, required requiredAny: PropTypes.any.isRequired,};
React Testing Library
This is a library for testing React, literally named "React Testing Library". It's also commonly referred to as RTL.
RTL grew in popularity due to its focus on testing functionality, not implementation details, as well as its inclusion in create-react-app.
Recently became popular over existing library Enzyme. Both libraries provide methods for rendering React components in tests, however RTL exposes functions that nudge developers away from testing implementation details.
Ref
Ref, short for reference, is used for accessing and modifying the child of a React component.
Usage:
function MyFunctionalComponent() { const myDiv = useRef(null);
return <div ref={myDiv} />;}
Particularly useful when integrating with legacy libraries that modify the DOM, and you just want to fetch a value from the DOM and continue using React.
Links: docs
RTL
Stateless Functional Component
How we used to call Functional Components before Hooks were added to React.
Basically, a stateless functional component is a functional component:
function Welcome(props) { return <h1>Hello, {props.name}</h1>;}
Styled Components
Styled Components is a library that allows developers to style their components by writing CSS in their JavaScript files (a solution known as CSS-in-JS).
TypeScript
A typed language that compiles to JavaScript, TypeScript rapidly checks types across your entire codebase at compile-time.
Now (optionally) included as part of CRA.
Links: cheatsheet | free book | handbook | website
webpack
webpack (with a lower-case w), is a program that reads through your codebase, follows your imports and exports, removes unused code, and outputs a bundle ready to be served by a server.
When reading webpack's docs, pay close attention to the version (in the top left hand corner). As the API greatly changes between major versions, you may think you've found a solution to your problems, but it may be unavailable in your version of webpack.
(Shameless plug for the useEffect book I wrote below)
Tired of infinite re-renders when using useEffect?
A few years ago when I worked at Atlassian, a useEffect bug I wrote took down part of Jira for roughly one hour.
Knowing thousands of customers can't work because of a bug you wrote is a terrible feeling. To save others from making the same mistakes, I wrote a single resource that answers all of your questions about useEffect, after teaching it here on my blog for the last couple of years. It's packed with examples to get you confident writing and refactoring your useEffect code.
In a single afternoon, you'll learn how to fetch data with useEffect, how to use the dependency array, even how to prevent infinite re-renders with useCallback.
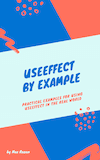